วันนี้มาตัว jsx Conditional Rendering เริ่มจาก Code ก่อนเลย
import './App.css' import PropTypes from 'prop-types'; const SelfIntroduction = ({name = "PingkungA", age, address}) => { return ( <div> <h3>My name is {name}</h3> {/* Conditional formatting by using short if (Ternary Operator) */} {age ? <h3>I'm {age} years old</h3> : null} {/* Conditional formatting by using if */} { (() => { console.log(address) if (address) { return ( <h3>I live in {address}</h3> ) } })() } </div> ) } SelfIntroduction.propTypes = { name: PropTypes.string.isRequired, age: PropTypes.number, address: PropTypes.string }; function App() { return ( <div className="App"> <SelfIntroduction name="PingkungA" age={33} address="Bangkok"/> </div> ) } export default App
แล้วมาดูผลลัพธ์กัน
- กรณีที่ส่งค่ามาครบ name / age / address แสดงผลหมด
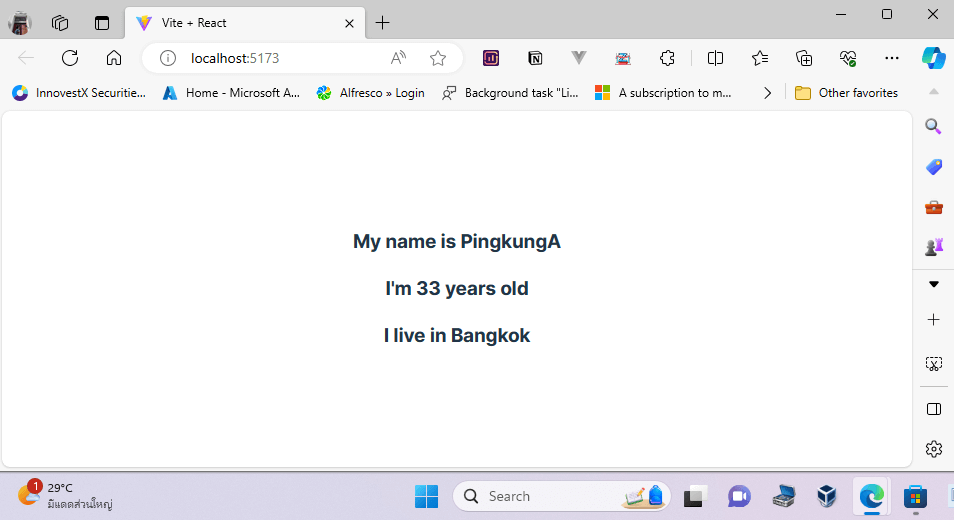
- กรณีที่ส่งค่ามาบางส่วน เช่น name ตัวอื่นๆจะถูกซ่อนไปหมด
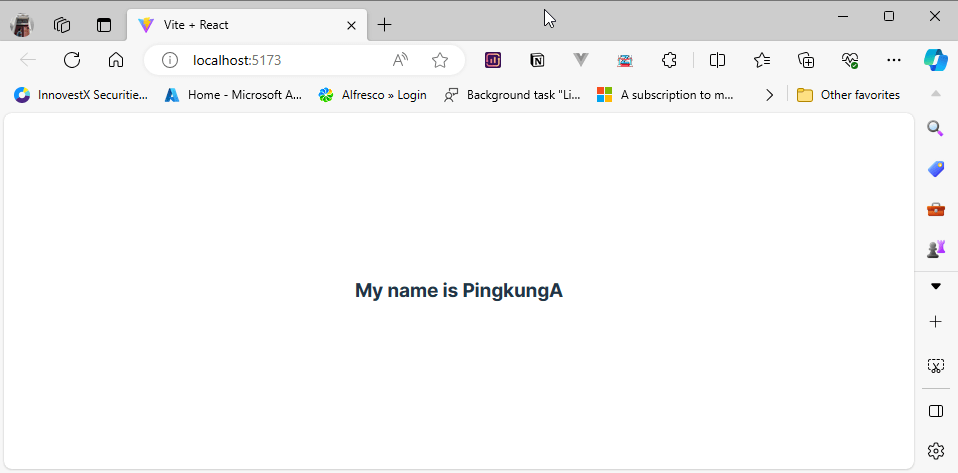
จริงๆ Conditional Rendering ทำได้ประมาณ 3-4 แบบนะ จาก Code ด้านบนผมตจะลองแบบ TERNARY operator / if เดี๋ยวลองมาดูจริงๆกันบ้าง
- Ternary Operator { Condition ? true action : false action }
const SelfIntroduction = ({name = "PingkungA", age, address}) => { return ( <div> <h3>My name is {name}</h3> {age ? <h3>I'm {age} years old</h3> : null} </div> ) }
- Logical operator (AND) ถ้าเงื่อนไขเป็นจริงแสดงผล ตัวอย่าง age มีค่าแสดงผลเลย
const SelfIntroduction = ({name = "PingkungA", age, address}) => { return ( <div> <h3>My name is {name}</h3> {age && <h3>I'm {age} years old</h3>} </div> ) }
- Logical operator (OR) ถ้ามีค่า name หรือ age เงื่อนไขจะเป็นจริง และแสดงค่าตัวแปรนั้น เคสนี้จะแสดง name
const SelfIntroduction = ({name = "PingkungA", age, address}) => { return ( <div> {name || age } </div> ) }
- if, else, else if
const SelfIntroduction = ({name = "PingkungA", age, address}) => { return ( <div> { (() => { if (!address) { return ( <h3>I live in somewhere.</h3> ) } else if (address === 'bangkok') { return ( <h3>I live in krung thep maha nakhon.</h3> ) } else { return ( <h3>I live in {address}.</h3> ) } })() } </div> ) }
- switch
const SelfIntroduction = ({name = "PingkungA", age, address}) => { return ( <div> { (() => { switch(true) { case (!address): { return ( <h3>I live in somewhere.</h3> ) } break; case (address === 'bangkok'): { return ( <h3>I live in krung thep maha nakhon.</h3> ) } break; default: { return ( <h3>I live in {address}.</h3> ) } break; } })() } </div> ) }
แบบ if, else, else if / switch จริงๆ มันคล้ายๆกันนะ แอบเปลืองด้วย ถ้ามีเคสจริงๆ คงใช้ Nested Ternary Operator
Discover more from naiwaen@DebuggingSoft
Subscribe to get the latest posts sent to your email.